Snippets
Browse collection of some of my coding snippets 😉
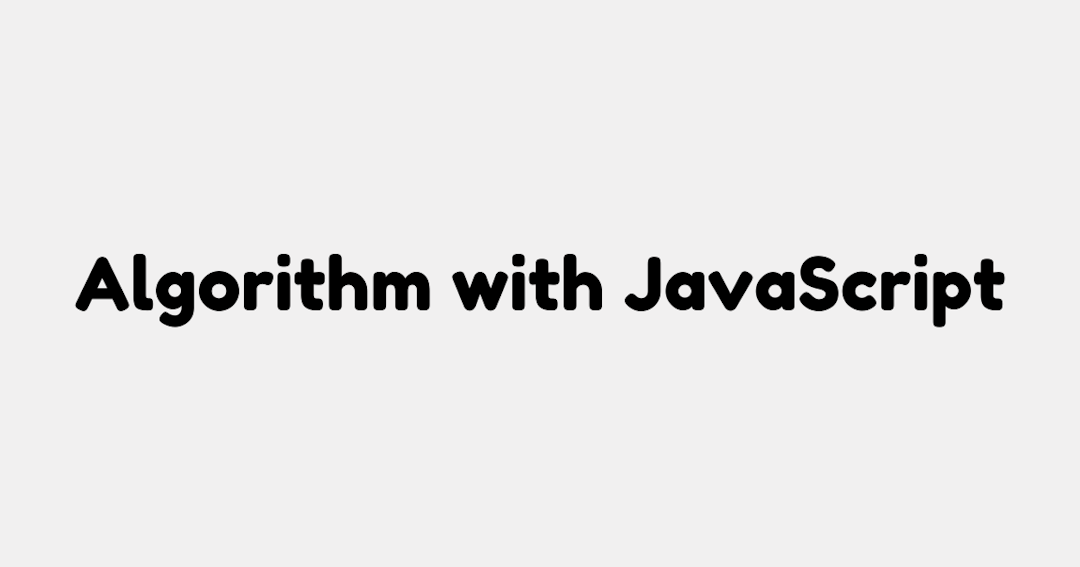
Array Intersection
Write a function called arrayIntersection that takes in two arrays and returns an array containing the intersection of the two input arrays (i.e., the common elements that appear in both arrays).
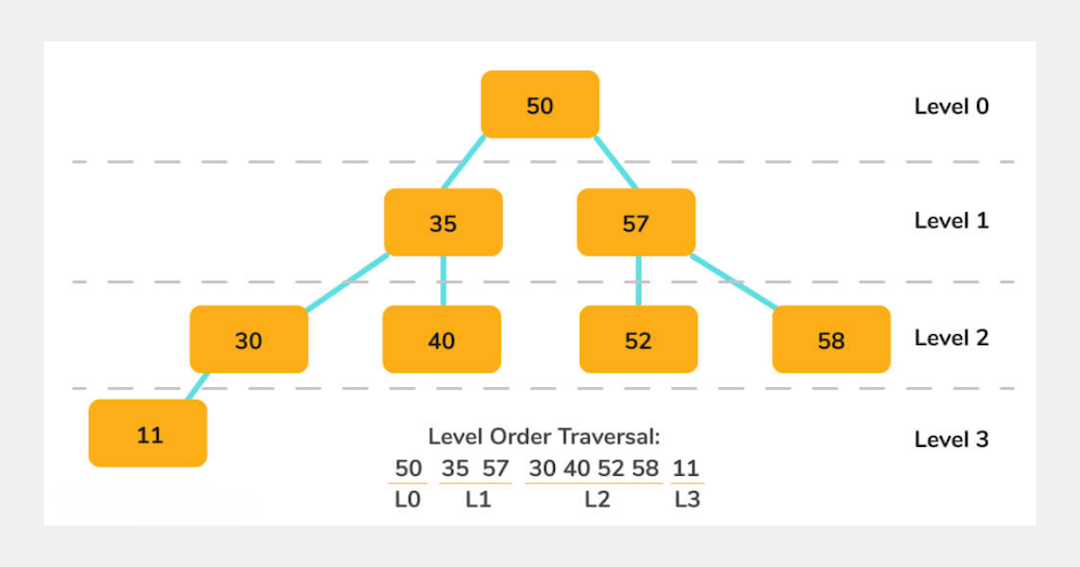
Binary Tree Level Order Traversal
Since we need to traverse all nodes of each level before moving onto the next level, we can use the Breadth First Search (BFS) technique to solve this problem.
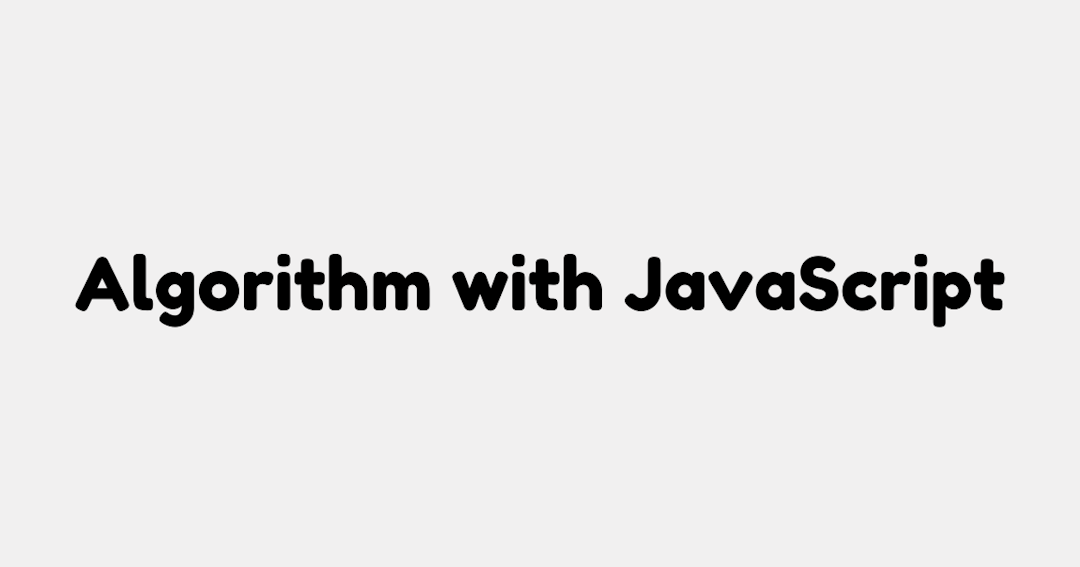
Calculate Power Using Recursion
The power of a number is the number multiplied to itself for the number of times it has been raised to
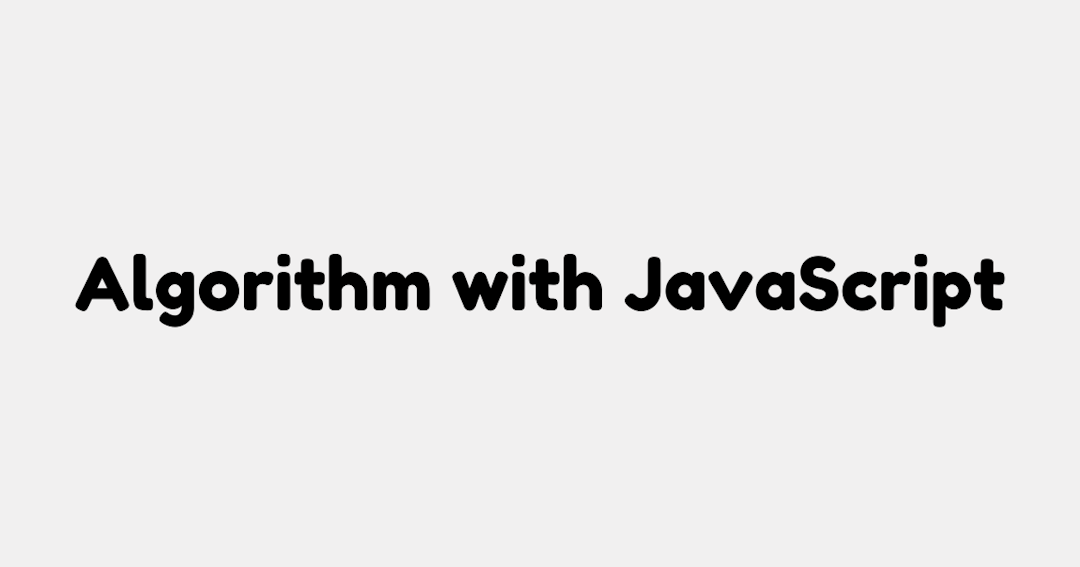
Calculator
Write a function called calculator that takes in 2 numbers and an operator and returns the result of the calculation.
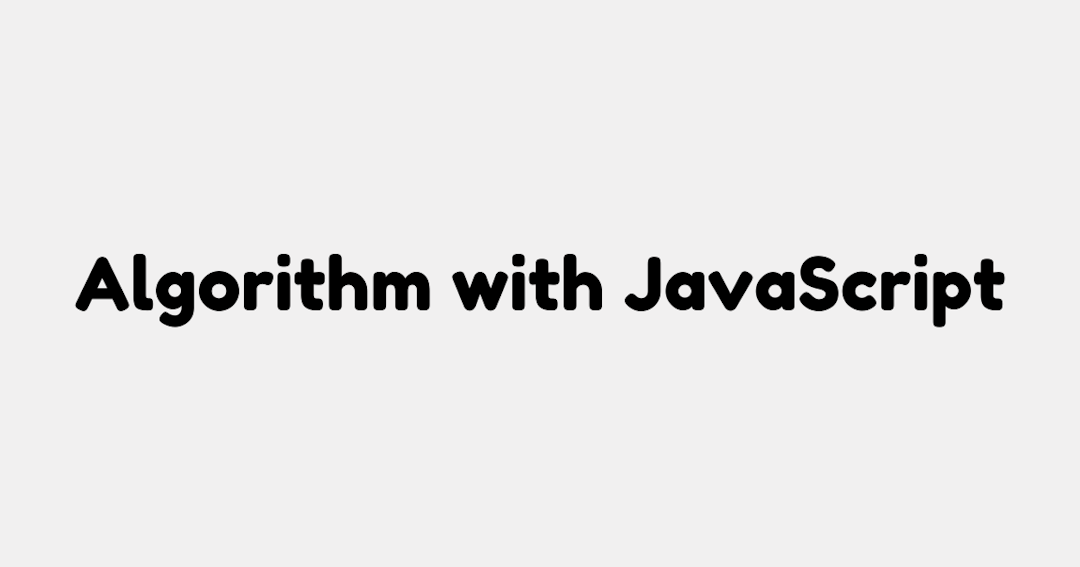
Count Occurrences
Write a function called countOccurrences() that takes in a string and a character and returns the number of occurrences of that character in the string.
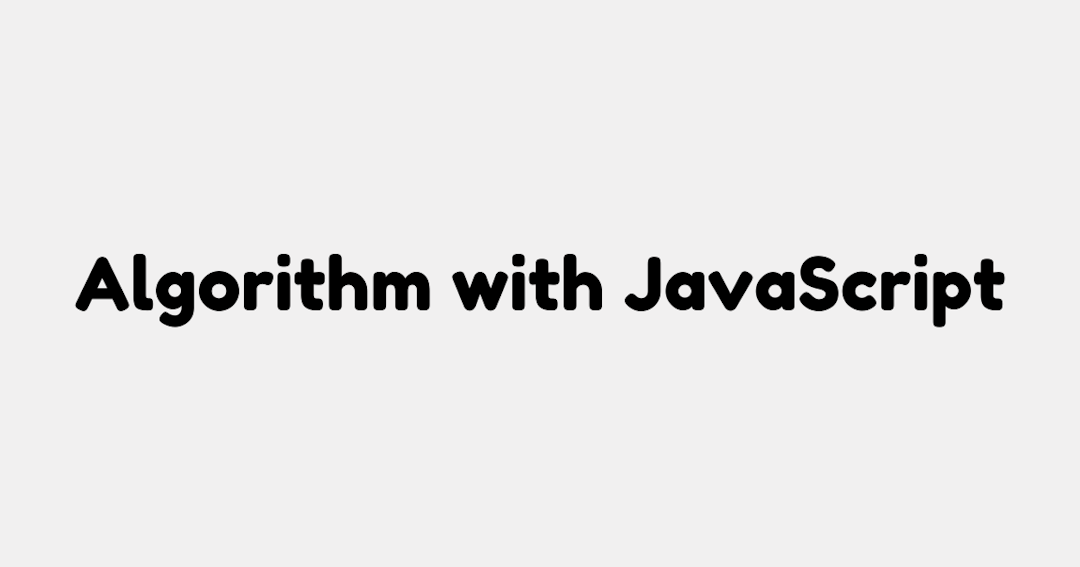
Factorial of Number Using Recursion
The factorial of a number is the product of all the numbers from 1 to that number.
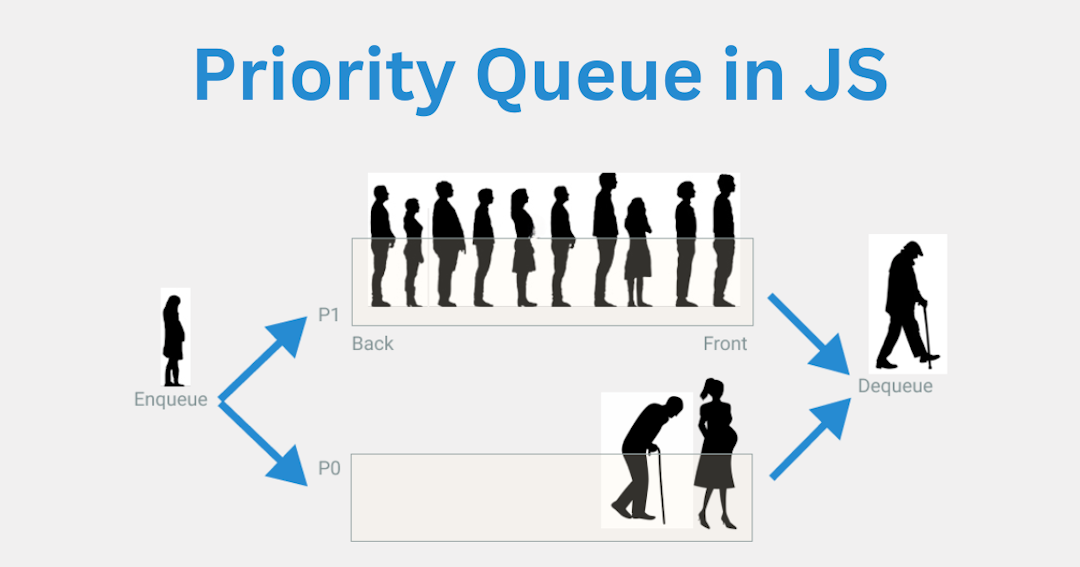
Kth Largest Element in an Array
MinHeap class and the findKLargestNumbers function implement a priority queue using a min-heap data structure.
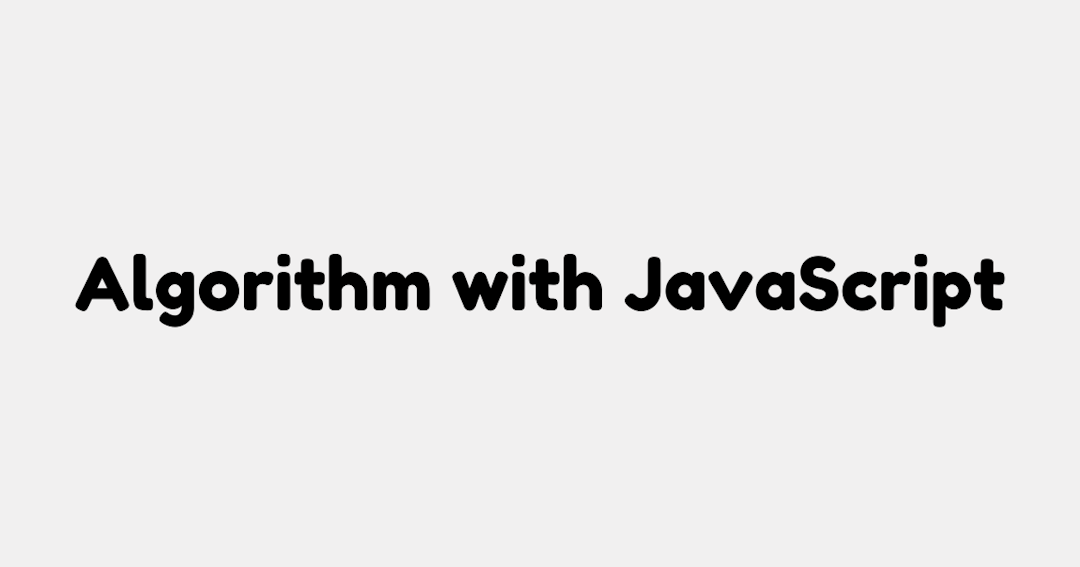
Program to Display Fibonacci Sequence Using Recursion
A Fibonacci sequence is the integer sequence of 0, 1, 1, 2, 3, 5, 8....
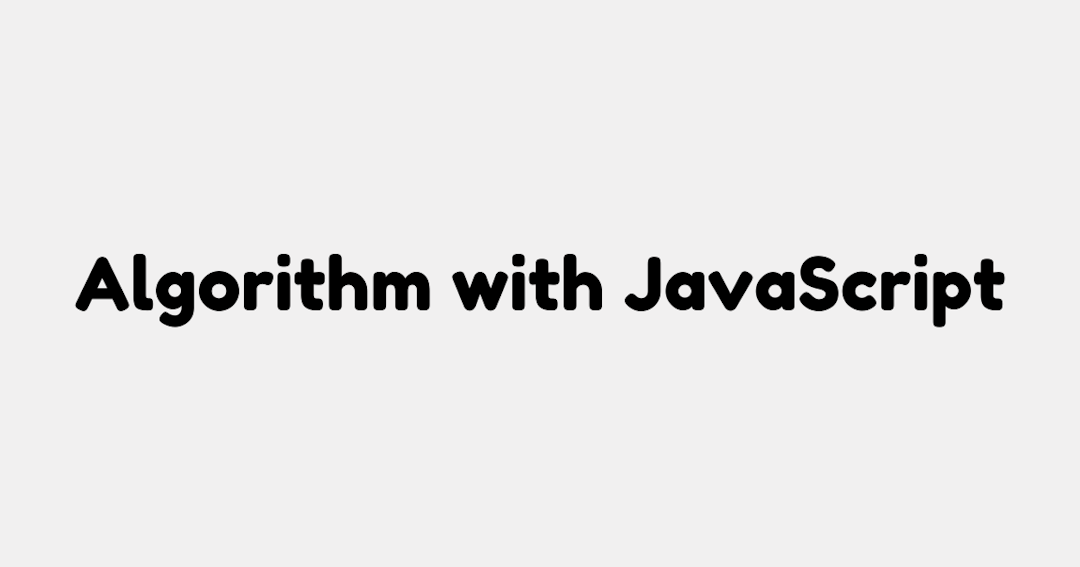
Title Case
Write a function called titleCase that takes in a string and returns the string with the first letter of each word capitalized.
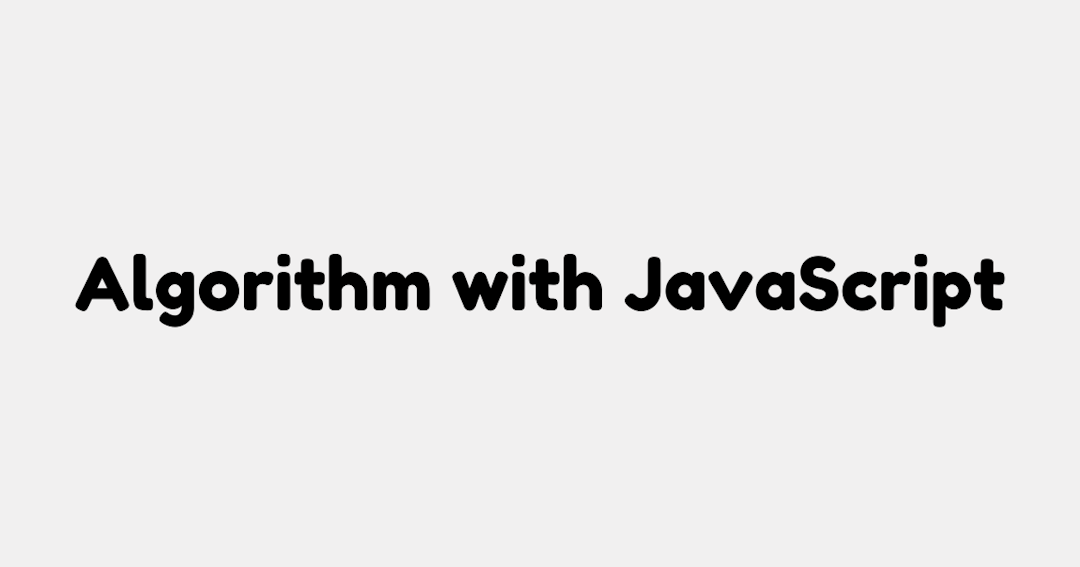
Topological Ordering
Topological Sort of a directed graph (a graph with unidirectional edges) is a linear ordering of its vertices such that for every directed edge (U, V) from vertex U to vertex V, U comes before V in the ordering.
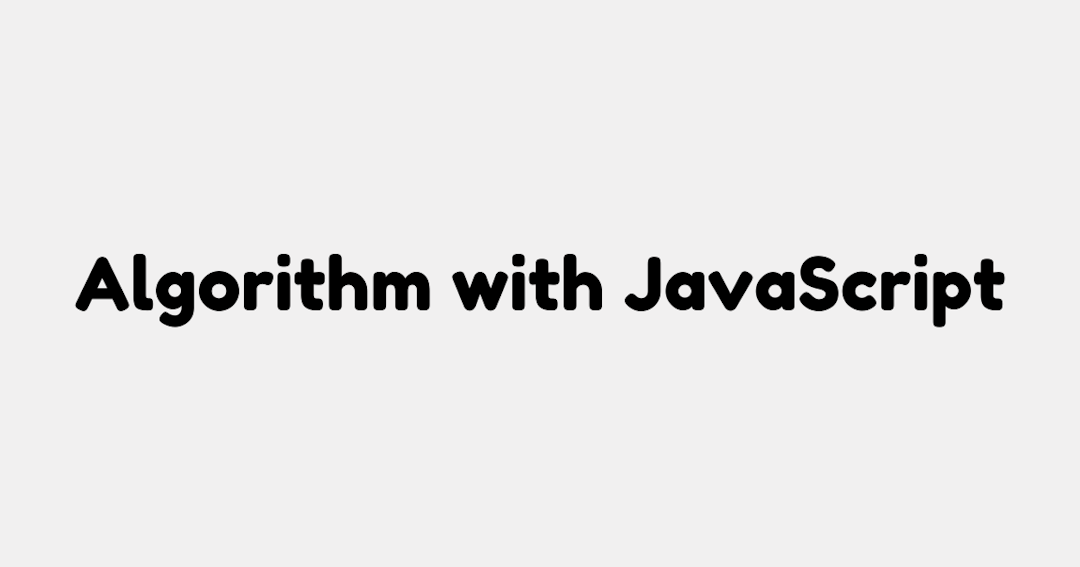
useLayoutEffect
Both of these can be used to do basically the same thing, but they have slightly different use cases.
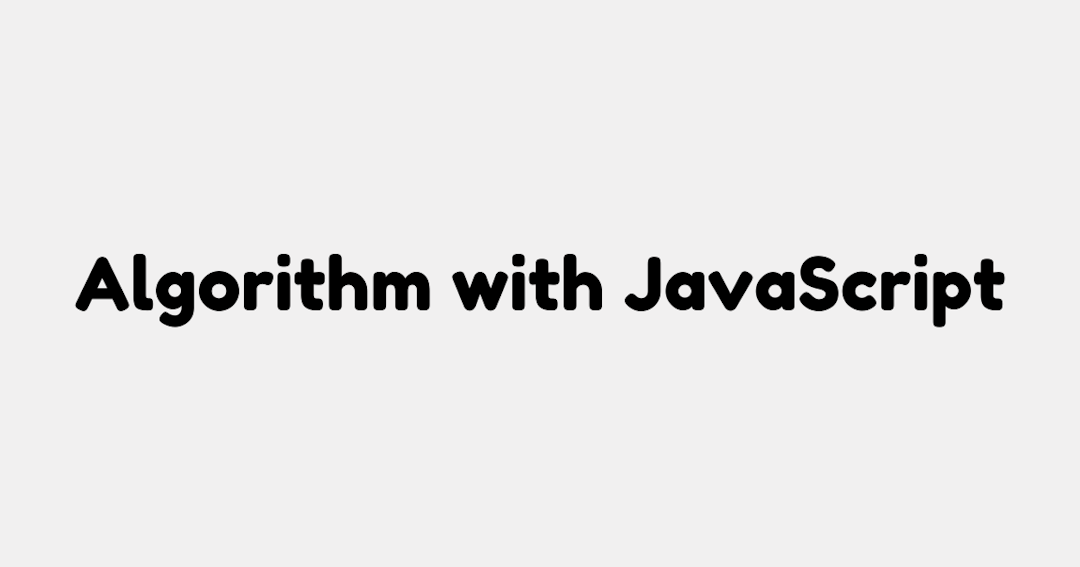
Using recursion to determine whether a word is a palindrome
A palindrome is a word that is spelled the same forward and backward. For example, rotor is a palindrome, but motor is not.